기본적으로 파이어베이스와의 연동이 다 끝난 상태라고 가정한 후 시작하겠습니다! 연동이 아직 안된 상태라면 파이어베이스와 연동을 끝낸 후 시작하시길 바랍니다
Firebase 클라우드 메시징 (FCM)
- 급하게 앱 서비스를 위해 푸쉬 알림이나 공지사항 등을 날릴 때 사용
- 기능: 알림 메시지 또는 데이터 메시지 전송, 다양한 메시지 타겟팅, 클라이언트 앱에서 메시지 전송
1. 권한 추가 - build.gradle
implementation 'com.google.firebase:firebase-messaging:21.0.1'
2. 서비스 설정 - AndroidManifest.xml
<service android:name=".MyFirebaseMsgService"
android:stopWithTask="false">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>
- <service>는 <activity> 아래에 추가해줍니다.
- service는 백그라운드에서 계속 작업되고 있는 것을 말합니다.
- service를 정의할 때는 자바 클래스로 정의합니다.
- intent-filter: 어떤 동작을 허용하겠다고 설정한 것입니다. 여기서는 메세지 이벤트를 받겠다고 설정했습니다.
- stopWithTask: 기본적으로 false로 설정해야 합니다. true를 하면 onTestRemoved가 수행되지 않고 갑자기 꺼졌을 때 에러 처리 등을 안한다는 의미입니다.
서비스(Service)의 종류
1. 포그라운드 서비스
- 앞 단에서 눈으로 보여주는 것
- 알림창에서 서비스가 실행중인 것을 표시해주는 형태
- 강제로 꺼지지는 않음, 눈에서 안보일 뿐 계속 실행 중
2. 백그라운드 서비스
- 사용자가 눈으로 못 보는 백 단에서 작업이 계속 돌고 있는 것
3. 바인드 서비스
- 서비스와 서비스를 호출하는 앱 구성요소가 서버-클라이언트 형태인 것
3. 서비스 정의 - MyFirebaseMsgService.java
public class MyFirebaseMsgService extends FirebaseMessagingService {
@Override
public void onMessageReceived(@NonNull RemoteMessage remoteMessage) {
if(remoteMessage != null && remoteMessage.getData().size() > 0) { //메시지의 텍스트가 있는지 확인
sendNotification(remoteMessage);
}
}
private void sendNotification(RemoteMessage remoteMessage) {
String title = remoteMessage.getData().get("title");
String msg = remoteMessage.getData().get("message");
final String CHANNEL_ID = "ChannerID";
NotificationManager mManager = (NotificationManager)getSystemService(Context.NOTIFICATION_SERVICE);
if(Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
final String CHANNEL_NAME = "ChannerName";
final String CHANNEL_DESCRIPTION = "ChannerDescription";
final int importance = NotificationManager.IMPORTANCE_HIGH;
// add in API level 26
// 빛, 진동패턴, 잠금 관련 내용 설정
NotificationChannel mChannel = new NotificationChannel(CHANNEL_ID, CHANNEL_NAME, importance);
mChannel.setDescription(CHANNEL_DESCRIPTION);
mChannel.enableLights(true);
mChannel.enableVibration(true);
mChannel.setVibrationPattern(new long[]{100, 200, 100, 200});
mChannel.setLockscreenVisibility(Notification.VISIBILITY_PRIVATE);
mManager.createNotificationChannel(mChannel);
}
NotificationCompat.Builder builder = new NotificationCompat.Builder(this, CHANNEL_ID);
builder.setSmallIcon(R.drawable.ic_launcher_background);
builder.setAutoCancel(true);
builder.setDefaults(Notification.DEFAULT_ALL);
builder.setWhen(System.currentTimeMillis());
builder.setSmallIcon(R.mipmap.ic_launcher);
builder.setContentTitle(title);
builder.setContentText(msg);
if(Build.VERSION.SDK_INT < Build.VERSION_CODES.O) {
builder.setContentTitle(title);
builder.setVibrate(new long[]{500, 500});
}
mManager.notify(0, builder.build());
}
@Override
public void onNewToken(@NonNull String s) {
super.onNewToken(s);
}
}
메시징 서비스를 사용하기 위해 FirebaseMessagingService를 상속 받습니다.
onMessageReceived( ) - 포그라운드 상태인 앱에서 알림 메시지 또는 데이터 메시지를 수신할 때 호출되는 메소드
remoteMessage - 전달받은 메시지
sendNotification( ) - 이 메소드는 전달받은 메시지를 커스텀화하는 부분입니다. 빛, 진동패턴 등을 설정할 수 있고, 알림 콘텐츠를 설정할 수 있습니다.
onNewToken( ) - 토큰을 설정하는 메소드입니다. 새로운 토큰이 생성될 때마다 호출됩니다. 단일 기기를 타겟팅하거나 기기그룹을 만들려면 이 메소드를 재정의해야 합니다.
4. 파이어베이스에서 알림 보내기
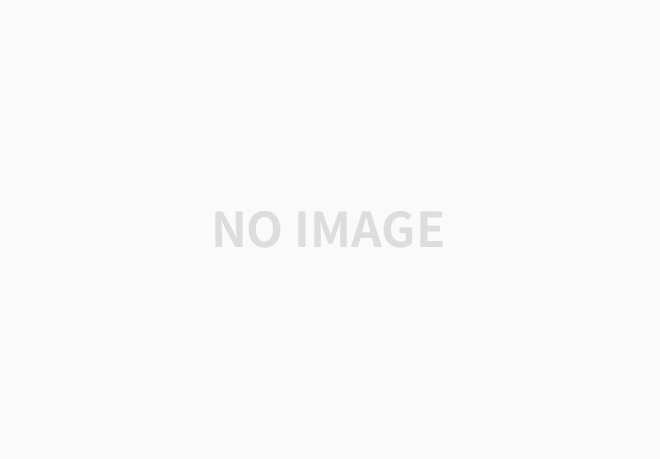
위와 같이 파이어베이스에서 알림 제목과 텍스트를 설정한 후 알림을 보내겠습니다.
알림을 보내면 다음과 같은 화면을 볼 수 있습니다.
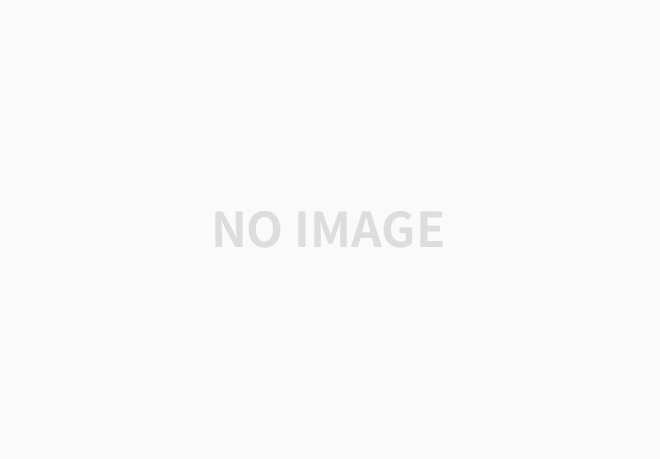
'Android' 카테고리의 다른 글
[Android] 12주차 스터디 (Volley) (0) | 2021.02.19 |
---|---|
[Android] 11주차 스터디 (Firebase Storage with Glide) (0) | 2021.01.27 |
[Android] 9주차 스터디 (Glide) (0) | 2021.01.07 |
[Android] 8주차 스터디 (Firebase) (1) | 2021.01.07 |
[Android] 네비게이션 드로어(Navigation Drawer) 사용하기 + 풀스크린(Fullscreen) (0) | 2020.12.27 |